Babylon.js
What is Babylon.js
Babylon.js is a JavaScript library for displaying 3D graphics in a web browser via HTML5. The source code is available on GitHub and distributed under the Apache License 2.0.
site : https://www.babylonjs.com/Exemplo de codigo : https://playground.babylonjs.com/
Exemplo de codigo : https://playground.babylonjs.com/#C21DGD#2
Modeling methodology.
The 3D modeling process used is that of polygon modeling with triangular faces to be represented by shell models. Limited use of constructive solid geometry is possible though only as a transitional method to create the union, subtraction and intersection of shell models. Once created models are rendered on an HTML 5 canvas element using a shader program which determines the pixel positions and colors on the canvas using the polygon models, the textures applied to each model, the scene camera and lights together with the 4 x 4 world matrices for each object which stores their position, rotation and scale. The technique used to produce photo realistic images is that of physically based rendering along with post-processing methods. In order to simulate collisions between models and other real world physical actions one of two physics engines need to be added as plugins, these are Cannon.js and Oimo. Animation involving, for example, changes in position or color of models is accomplished by key frame animation objects called animatables, while full character animation is achieved through the use of skeletons with blend weights.
// This creates a basic Babylon Scene object (non-mesh)
var scene = new BABYLON.Scene(engine);
// This creates and positions a free camera (non-mesh)
var camera = new BABYLON.FreeCamera("camera1", new BABYLON.Vector3(0, 5, -10), scene);
// This targets the camera to scene origin
camera.setTarget(BABYLON.Vector3.Zero());
// This attaches the camera to the canvas
camera.attachControl(canvas, true);
// This creates a light, aiming 0,1,0 - to the sky (non-mesh)
var light = new BABYLON.HemisphericLight("light", new BABYLON.Vector3(0, 1, 0), scene);
// Default intensity is 1. Let's dim the light a small amount
light.intensity = 0.7;
// Our built-in 'sphere' shape.
var sphere = BABYLON.MeshBuilder.CreateSphere("sphere", {diameter: 2, segments: 32}, scene);
// Move the sphere upward 1/2 its height
sphere.position.y = 1;
// Our built-in "ground" shape.
Our built-in "ground" shape.
var ground = BABYLON.MeshBuilder.CreateGround("ground", {width: 6, height: 6}, scene);
return scene;
};
RENDERER
mais informação em breve ...
Geometrias 3D
Box Mesh
The created box has its origin at the center of the box, its width (x), height (y) and depth (z) are as given.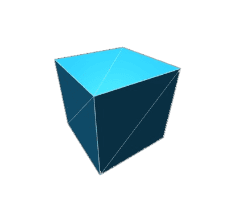
Sphere
The created sphere has its origin at the center of the sphere. By using different values for diameterX, diameterY and diameterZ_ lead you create an ellipsoid.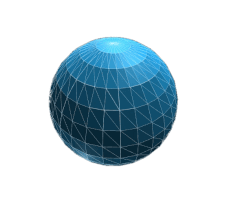
//scene is optional and defaults to the current scene.
Cone
If you set diameterTop to zero, you get a cone instead of a cylinder, with different values for diameterTop and diameterBottom you get a truncated cone.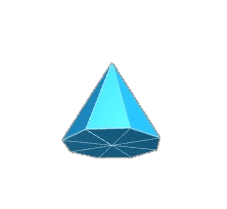
//scene is optional and defaults to the current scene.
Cylinder
The created cylinder has its flat sides parallel to the xz plane with its origin at the center of the vertical line of symmetry. If you set diameterTop to zero, you get a cone instead of a cylinder, with different values for diameterTop and diameterBottom you get a truncated cone.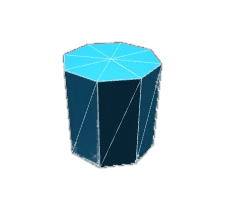
const cylinder = BABYLON.Mesh.CreateCylinder("cylinder", height, diameterTop, diameterBottom, tessellation, subdivisions, scene, updatable, sideOrientation);
//optional parameters after scene
Torus
The created torus (doughnut shape) has its origin at the center of the torus. You can control its diameter and the thickness of its circular body.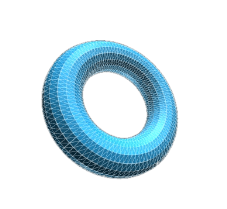
const torus = BABYLON.Mesh.CreateTorus("torus", diameter, thickness, tessellation, scene, updatable, sideOrientation);
//optional parameters after scene
TorusKnot
A torus knot is a continuous shape that twists and turns around the surface of a torus. The number of twists and turns are determined by two windings integers p and q. The simplest knotted knot is with 2 and 3 for p and q. The origin of the created torus knot is at the center of the underlying torus.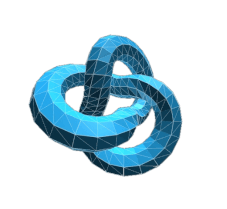
const knot = BABYLON.Mesh.CreateTorusKnot("knot", radius, tube, radialSegments, tubularSegments, p, q, scene, updatable, sideOrientation);
// Optional parameters after scene.